# back-stage management
# The following commands are run in the project root directory
# Base page
- Renderings
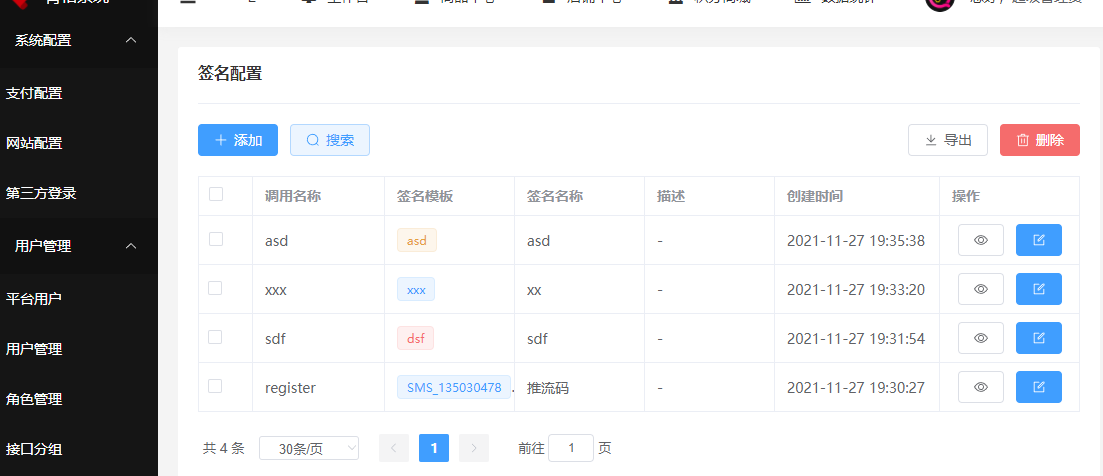
- Laravel
# Generate the corresponding controller file. The model file needs to be placed in the [qingwuit/model] directory
php artisan make:controller Admin/xxxController # Seller/xxxController
class NoticesController extends Controller
{
protected $modelName = 'Notice'; #Corresponding to the model file generated by laravel
protected $setUser = true; # Whether to distinguish the users. Default false
}
- Vue
<!-- resources\js\views Create a new file under the directory Vue -->
<!-- Example:views\Admin\notices\index.vue -->
<template>
<table-view :options="options" :searchOption="searchOptions" :dialogParam="dialogParam"></table-view>
</template>
<script>
import {reactive,getCurrentInstance} from "vue"
import tableView from "@/components/common/table"
export default {
components:{tableView},
setup(props) {
const {ctx,proxy} = getCurrentInstance()
// List column contents
const options = reactive([
{label:'标题',value:'name'},
{label:'标签',value:'tag',type:"tags"},
{label:'创建时间',value:'created_at'},
]);
// Search field
const searchOptions = reactive([
{label:'标题',value:'name',where:'likeRight'},
{label:'标签',value:'tag',where:'likeRight'},
{label:'内容',value:'content',where:'like'},
])
// Form configuration
const addColumn = [
{label:'标题',value:'name'},
{label:'标签',value:'tag'},
{label:'内容',value:'content',type:'editor',span:24,viewType:'html'},
]
const dialogParam = reactive({
rules:{
name:[{required:true,message:'不能为空'}]
},
view:{column:addColumn},
add:{column:addColumn},
edit:{column:addColumn},
})
return {options,searchOptions,dialogParam}
}
}
</script>
# Custom table list
<template>
<table-view :options="options" >
<template #custom_item="row">
<!-- Custom content area -->
</template>
</table-view>
</template>
<script>
import {reactive} from "vue"
import tableView from "@/components/common/table"
export default {
components:{tableView},
setup() {
// List column contents
const options = reactive([
{label:'Custom list',value:'custom_item',type:"custom"},
]);
return {options}
}
}
</script>
# Other configuration APIs
<template>
<table-view :options="options" :pagination="pagination" :handleWidth="handleWidth" :handleHide="handleHide" :params="params" :searchOption="searchOptions" :btnConfig="btnConfigs" :dialogParam="dialogParam">
<template #custom_item="row">
<!-- Custom content area -->
</template>
</table-view>
</template>
<script>
import {reactive,ref} from "vue"
import tableView from "@/components/common/table"
export default {
components:{tableView},
setup() {
// 列表列内容
const options = reactive([
{label:'Custom content area',value:'custom_item',type:"custom"},
]);
// This parameter controls the display of the add / delete / modify query button
const btnConfigs = reactive({
show:{show:true}, // show
store:{show:true}, // store
update:{show:true}, // update
destroy:{show:true}, // destroy
deletes:{show:false}, // Delete single line
search:{show:true}, // search
export:{show:true},
import:{show:false},
})
const dialogParam = reactive({
// Dictionary, displaying dictionary contents according to key values
dictData:{
status:[{label:proxy.$t('btn.waitUse'),value:0},{label:proxy.$t('btn.used'),value:1}]
},
width:'50%', // Bullet frame size
labelWidth:'90px', // form label Font width
span:12, // Item Default width
column:[], // Default field
fullscreen:false, // Full screen
rules:null,
destroyOnClose:true,
isPageDict:false,
dict:[], // Dictionary links {name:"menus",url:'xxx.com'}
addOpenBefore:()=>{}, // Execute before opening add
editOpenBefore:()=>{}, // Execute before opening edit
editOpenAfter:()=>{},
add:{
column:[], // {label:'My game',value:'name'},{label:'Location key',value:'name'},{label:'Avatar',value:'name'}
},
edit:{
column:[],
},
view:{
column:[],
}
})
const handleWidth = ref('120px') // Operation column width
const handleHide = ref(true) // Whether the action column is displayed
const pagination = ref(true) // Show Pagination
const pageUrl = ref('') // The URL of the query list defaults to the current URL, which is customized here
// List displayed condition query
const params = reactive({
is_belong:'0|gt',
})
return {options,btnConfigs,dialogParam,params,handleWidth,handleHide,pagination,pageUrl,handleHide}
}
}
</script>
# Custom page
- Vue
<template>
<!-- chat -->
<base-view>
<template #main_view>
<chat ref="chat" :closeVis="false" :params="data.chatParams" />
</template>
</base-view>
</template>
<script>
import {reactive,onMounted,nextTick,getCurrentInstance} from "vue"
import Chat from "@/components/common/chat"
import baseView from "@/components/common/base"
export default {
components:{baseView,Chat},
setup(props) {
const {proxy} = getCurrentInstance()
const data = reactive({
chatParams:{provider:'users',rid:null,rtype:null,token:localStorage.getItem('seller_token')}
})
onMounted(()=>{
proxy.$refs.chat.openChat()
})
return {
data,
}
}
}
</script>
<style lang="scss" scoped>
.chat_main{
height: 600px;
}
</style>