# 后台管理
# 以下命令都运行在项目根目录
# 基础页面
- 效果图
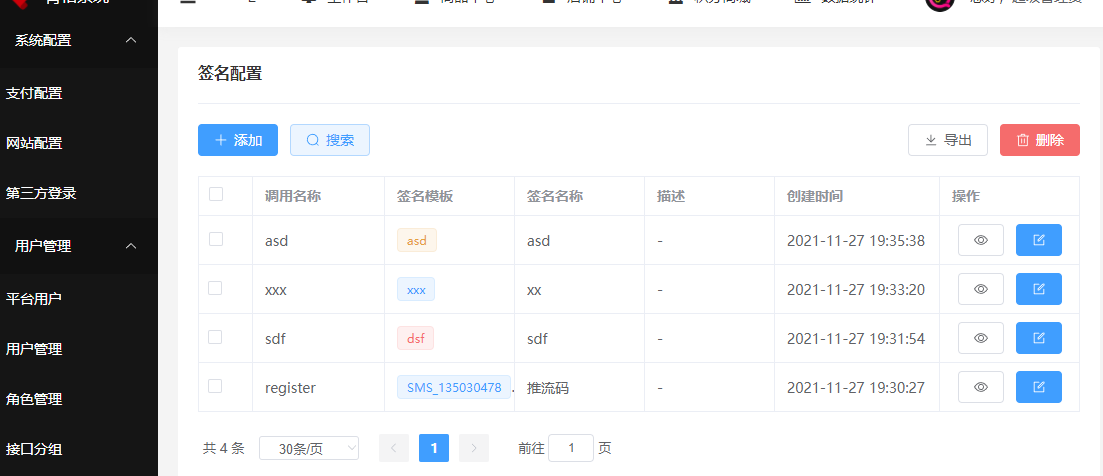
- Laravel
# 生成对应的控制器文件 Model文件需要放入 [Qingwuit/Model] 目录下
php artisan make:controller Admin/xxxController # Seller/xxxController
class NoticesController extends Controller
{
protected $modelName = 'Notice'; #对应Laravel 生成的 Model文件
protected $setUser = true; # 是否区分所属用户默认False
}
- Vue
<!-- resources\js\views 目录下新建文件你自己vue -->
<!-- 示例:views\Admin\notices\index.vue -->
<template>
<table-view :options="options" :searchOption="searchOptions" :dialogParam="dialogParam"></table-view>
</template>
<script setup>
import {reactive,getCurrentInstance} from "vue"
import tableView from "@/components/common/table"
const {proxy} = getCurrentInstance()
// 列表列内容
const options = reactive([
{label:'标题',value:'name'},
{label:'标签',value:'tag',type:"tags"},
{label:'创建时间',value:'created_at'},
]);
// 搜索字段
const searchOptions = reactive([
{label:'标题',value:'name',where:'likeRight'},
{label:'标签',value:'tag',where:'likeRight'},
{label:'内容',value:'content',where:'like'},
])
// 表单配置
const addColumn = [
{label:'标题',value:'name'},
{label:'标签',value:'tag'},
{label:'内容',value:'content',type:'editor',span:24,viewType:'html'},
]
const dialogParam = reactive({
rules:{
name:[{required:true,message:'不能为空'}]
},
view:{column:addColumn},
add:{column:addColumn},
edit:{column:addColumn},
})
</script>
# 自定义Table列表
<template>
<table-view :options="options" >
<template #custom_item="row">
<!-- 自定义内容区域 -->
</template>
</table-view>
</template>
<script setup>
import {reactive} from "vue"
import tableView from "@/components/common/table"
// 列表列内容
const options = reactive([
{label:'自定义列表',value:'custom_item',type:"custom"},
]);
</script>
# 其他配置API
<template>
<table-view :options="options" :pagination="pagination" :handleWidth="handleWidth" :handleHide="handleHide" :params="params" :searchOption="searchOptions" :btnConfig="btnConfigs" :dialogParam="dialogParam">
<template #custom_item="row">
<!-- 自定义内容区域 -->
</template>
</table-view>
</template>
<script setup>
import {reactive,ref} from "vue"
import tableView from "@/components/common/table"
// 列表列内容
const options = reactive([
{label:'自定义列表',value:'custom_item',type:"custom"},
]);
// 这个参数控制 增删改查按钮的显示
const btnConfigs = reactive({
show:{show:true}, // 显示
store:{show:true}, // 添加
update:{show:true}, // 编辑
destroy:{show:true}, // 删除
deletes:{show:false}, // 删除单行
search:{show:true}, // 搜索
export:{show:true},
import:{show:false},
})
const dialogParam = reactive({
// 字典,根据键值 显示字典内容
dictData:{
status:[{label:proxy.$t('btn.waitUse'),value:0},{label:proxy.$t('btn.used'),value:1}]
},
width:'50%', // 弹框大小
labelWidth:'90px', // form label 字体宽度
span:12, // Item 默认宽度
column:[], // 默认字段
fullscreen:false, // 是否全屏
rules:null,
destroyOnClose:true,
isPageDict:false,
dict:[], // 字典链接 {name:"menus",url:'xxx.com'}
addOpenBefore:()=>{}, // 打开添加之前执行
editOpenBefore:()=>{}, // 打开编辑之前执行
editOpenAfter:()=>{},
add:{
column:[], // {label:'我的游戏',value:'name'},{label:'定位密钥',value:'name'},{label:'头像',value:'name'}
},
edit:{
column:[],
},
view:{
column:[],
}
})
const handleWidth = ref('120px') // 操作列宽度
const handleHide = ref(true) // 操作列是否显示
const pagination = ref(true) // 显示分页
const pageUrl = ref('') // 查询列表的url 默认当前url 这里是自定义的
// 列表显示的条件查询
const params = reactive({
is_belong:'0|gt',
})
</script>
# 自定义页面
- Vue
<template>
<!-- 聊天 -->
<base-view>
<template #main_view>
<chat ref="chat" :closeVis="false" :params="data.chatParams" />
</template>
</base-view>
</template>
<script setup>
import {reactive,onMounted,nextTick,getCurrentInstance} from "vue"
import Chat from "@/components/common/chat"
import baseView from "@/components/common/base"
const {proxy} = getCurrentInstance()
const data = reactive({
chatParams:{provider:'users',rid:null,rtype:null,token:localStorage.getItem('seller_token')}
})
onMounted(()=>{
proxy.$refs.chat.openChat()
})
</script>